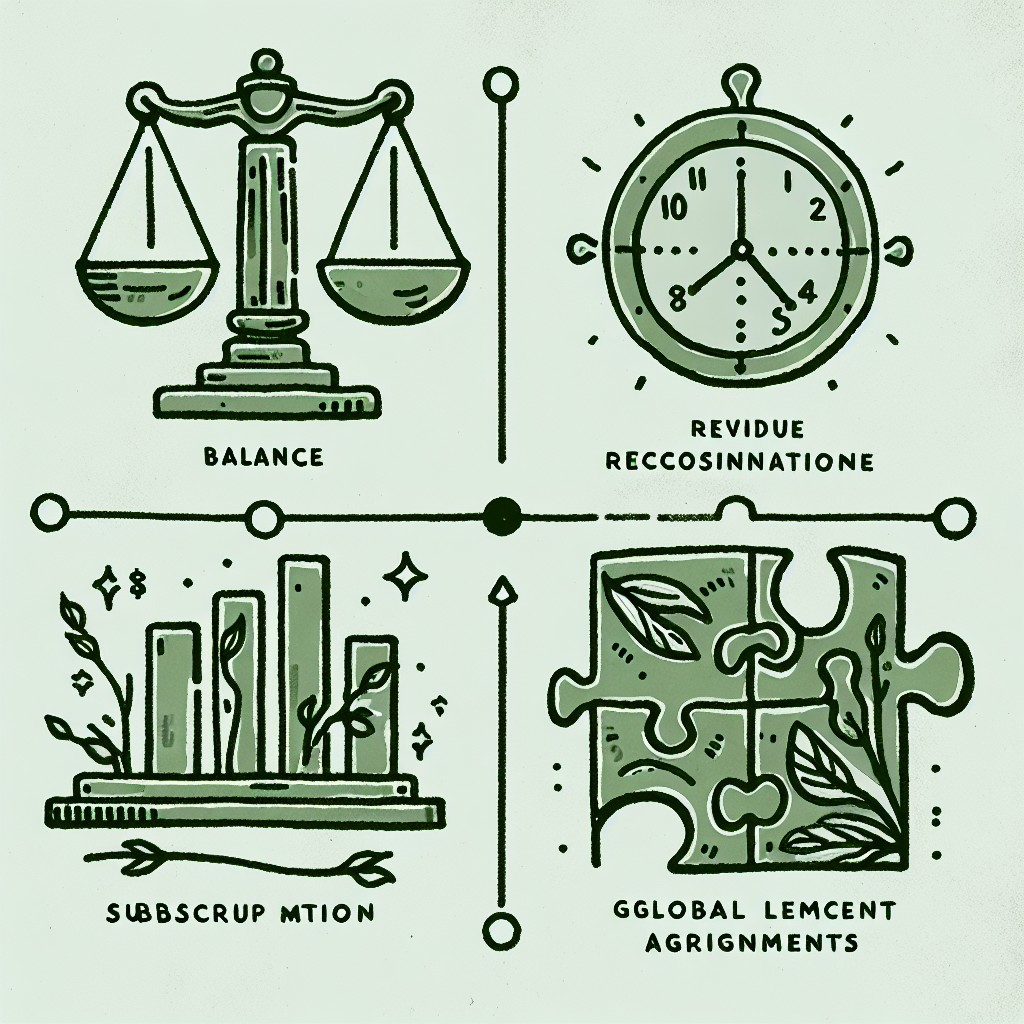
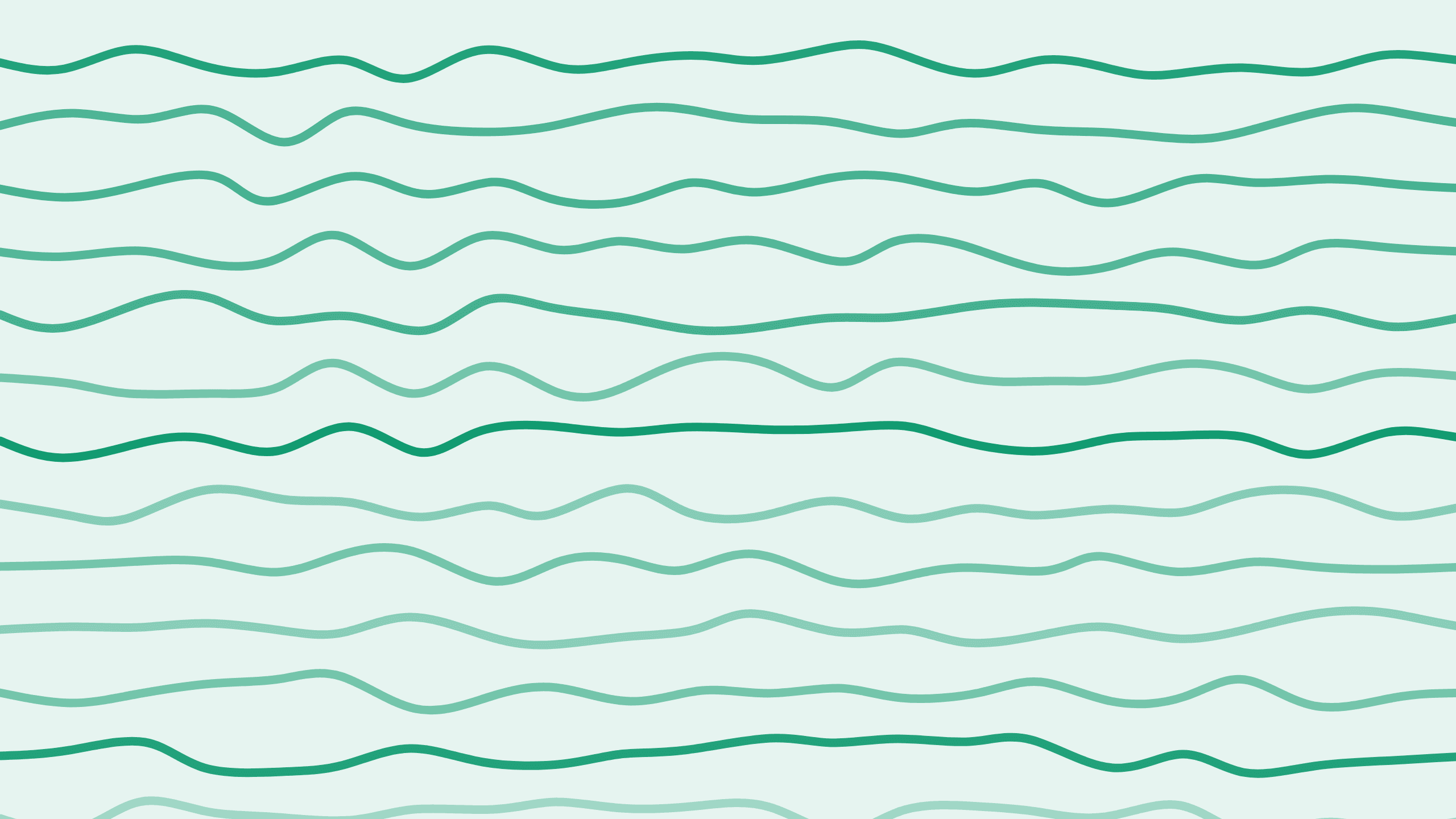
Localized Pricing with Paddle: A Comprehensive Guide
Hi, I'm Alex and welcome back to the Boathous Paddle Series where we discuss all things related to Paddle. In today's post, we're going to dive into how to integrate pricing information from Paddle into your marketing website, specifically into your pricing table. This builds on an example from a previous video where we set up a demo product page with Paddle checkout.
What You'll Learn
In this post, we will:
- Set up localized pricing on your website using Paddle
- Implement the necessary JavaScript to display the correct price
- Handle automatic currency conversions
- Use a third-party service for IP address localization
Setting the Scene
Imagine you have a product page with a Paddle checkout already running. This is great, but you also want to display the price directly on your webpage. Not just the base price you've set up in Paddle, but also the localized pricing reflecting currency conversions or country-specific overrides.
Here's a step-by-step guide to achieve this:
Step 1: Head Over to Carrd
First, we'll navigate to card, our website builder for this example.
-
Adding a Text Field:
- Add a text field and give it an ID of
price
. This ID allows us to reference it later in our JavaScript. - Example Code:
- Add a text field and give it an ID of
<span id="price"></span>
-
Setting Up JavaScript:
- The JavaScript example we'll use is quite simple. The upper section sets up your Paddle environment, including switching to the sandbox environment and adding a client-side token.
Paddle.Setup({
vendor: YOUR_VENDOR_ID,
environment: "sandbox",
// add your client-side token
})
Step 2: Fetching the Price Data
The core of displaying the correct price involves using Paddle's price preview function.
-
The Price Preview Function:
- Pass in the product's price ID with a quantity to get the price.
Paddle.Price({ product_id: YOUR_PRODUCT_ID, quantity: 1 })
-
Handling Localization:
- Retrieve the user's IP address to determine their location and adjust the price accordingly.
fetch("https://api.ipify.org?format=json") .then((response) => response.json()) .then((data) => { const ipAddress = data.ip Paddle.Price({ product_id: YOUR_PRODUCT_ID, quantity: 1, customer_ip: ipAddress, success: function (price) { document.getElementById("price").innerText = price.formatted }, }) })
Step 3: Dealing with Automatic Currency Conversions
Paddle allows automatic currency conversions, essential for presenting localized pricing without manual updates.
-
Implementation in JavaScript:
- The price preview function will take the IP address and provide the localized currency, including the correct symbol and format.
function getLocalizedPrice(productId, ipAddress) { Paddle.Product.Price(productId, { customer_ip: ipAddress, success: function (price) { document.getElementById("price").innerText = price.formatted }, }) }
-
Flexibility for Multiple Products or Bundles:
- If your pricing table includes multiple products or has a quantity selector, you can adjust the preview function to reflect these complexities.
Paddle.Price({ product_id: YOUR_PRODUCT_ID, quantity: SELECTED_QUANTITY, success: function (price) { document.getElementById("price").innerText = price.formatted }, })
Step 4: Integrating the IP Address Service
Using a third-party service like IPI is crucial to get the user's IP address for localized pricing.
-
Fetching the IP Address:
fetch("https://api.ipify.org?format=json") .then((response) => response.json()) .then((data) => { const ipAddress = data.ip getLocalizedPrice(YOUR_PRODUCT_ID, ipAddress) })
Potential Issues and Workarounds
What About VPNs?
VPNs can create some challenges by masking the true location of the user, which may lead to incorrect pricing display.
-
Testing VPN Use:
- For example, switching to a Canadian VPN should show a price in Canadian Dollars if set up correctly.
"What happens if I use a VPN to put myself into a different country?"
-
Business to Business Concerns:
- If a business uses a VPN to show a different country but needs an invoice from their actual country, the system will correct the price to the local currency once they try to finalize the purchase and add business details.
Handling Real-time Changes
On some occasions, users might experience a brief flash while the website fetches updated pricing.
-
Fallback Solution:
- Display a standard price (e.g., in dollars) immediately and update it once the localized price is fetched.
document.getElementById("price").innerText = "$50" // Default pricefetchLocalizedPrice();
Conclusion
Incorporating localized pricing into your website using Paddle is straightforward when you follow these steps. By leveraging Paddle's API and third-party IP address services, you can ensure your users see the most relevant pricing right away.
If you have any questions or run into issues, feel free to leave a comment or reach out directly.
Happy coding!
Using localized pricing not only enhances user experience but also increases conversion rates by presenting relevant information seamlessly.
Stay tuned for our next post where we will explore more advanced features of Paddle and how you can use them to optimize your checkout process. Talk to you soon!